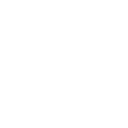
Practical experience of making HTTP driver
This theme is based on iRidium DDK. Below is the example of making driver for almost any device that has a web interface.
To make a driver you will need the Device that is connected to your LAN and has static IP address and LAN sniffer on your PC (in my case it is WireShark).
1. Getting request for feedbacks of the Device
- start your browser
- start your sniffer
- go to the web page of your device (in my case 192.168.1.11) or update it
- in sniffer window you should now find request from your PC to the Device
This URI is needed for us request for getting feedback of device (feedback is all the data that is on selected page.
method: GET
URI: /MainZone/index.html
2. Getting feedback
in JS if you make a request:
var DEVICE = IR.GetDevice("Busch iNet Radio");
DEVICE.Send(['GET,/en/index.shtml']);
as the answer you will get full HTTP code of the page you were requesting.
JS getting feedback:
IR.AddListener(IR.EVENT_RECEIVE_TEXT, DEVICE, function(inText)
{IR.Log(inText);}
LOG:
<html>
<head>
<title>Internet-Radio</title>
<link href="/style/style.css" type="text/css"/>
</head>
<body >
.........
<legend>Volume</legend>
<p>
<input type="text" name="vo" size=5 maxlength=5 value="2" />..........
<legend>Currently playing</legend>
<p>
Station 5:
<p>
<input type="text" name="--" readonly size=70 maxlength=64 value="DI.fm | Vocal chillout" />
..........
3. Parsing feedback text
To get needed data you have to slice this text.
For volume it will be:
var volume3 = inText.slice(inText.indexOf("Volume", 0)+60,inText.indexOf("Volume", 0)+120);
//getting part of the text for volume
var volume2 = volume3.slice(volume3.indexOf("value=", 0)+7,volume3.indexOf("nbsp", 0)-6);
//getting value
var volume = parseFloat(volume2);
//making float from text
IR.SetVariable("Drivers."+DeviceName+".iNet_Volume",volume);
//writing to variable
The same for current station:
var station0_2 = inText.slice(inText.indexOf("Currently playing", 0)+30,inText.indexOf("Currently playing", 0)+250);
var station0 = station0_2.slice(station0_2.indexOf("value=", 0)+7,station0_2.indexOf("Add to TuneIn Presets", 0)-71);
IR.SetVariable("Drivers."+DeviceName+".iNet_Current station name",station0);
This should be done for every data you need.
4. How to get HTTP command from the page
The same way that described in #1 but instead of updating page you have to press needed button.
In my case it is:
method: GET
URI: /en/index.cgi?p1=+Play+
JS:
DEVICE.Send(['GET,/en/index.cgi?p1=+Play+']);
The only thing to do is to ling this with buttons.
5. Cicling request for feedback
Data should periodically be updated. To do this:
function Autorequest()
{
DEVICE.Send(['GET,/en/index.shtml']);
}
IR.AddListener(IR.EVENT_ITEM_SHOW,IR.GetItem(Page),function() //getting feedback when page is shown
{
var ID = IR.SetInterval(5000, Autorequest); //every 5s
});
IR.AddListener(IR.EVENT_ITEM_HIDE,IR.GetItem(Page),function()
{
IR.ClearInterval(ID); //Need to be cleared. If not it will bee starting new interval request which will make your connection down
});
In attach example project and page HTTP code
This could be done for every device with web interface: UPS, Radio, etc.
Hope this will be helpfull for you
:)
Customer support service by UserEcho
Thank you!
This should go to FAQ for driver development.
thank you for sharing this..