Send a command to Global Cache from the script
SEND COMMAND
If the command is already created in the device tree (built-in drivers):
http://wiki2.iridiummobile.net/Drivers_API#Set
If you want to form the command string inside the script:
http://wiki2.iridiummobile.net/Drivers_API#Send
Take part in webinars of iRidium Academy to receive more information about working with equipment using iRidium Script.
Let’s say you have GC-100-12, and some RS232-Device is connected to the output Serial 2. RS232-Device has a set of commands described in the driver (in output Commands).
So in order to activate the command what should you write?
One GC-100-12 module consists of 3 transports (as it works with 3 different devices at a time):
- port 4998 – IR commands, system data, relay control
- port 4999 – Serial 1
- port 5000 – Serial 2
Thus, to refer to different ports, you will need to describe the device differently from the standard AV driver.
.Send command to GC:
IR.GetDevice("Global Cache").Send(['DATA'], <TRANSPORT ID>)
is 0, 1 or 2 - it is the number of TCP transport created by GC driver
0 = TCP transport on port 4998 (IR commands, system data, relays)
1 = TCP transport on port 4999 (Serial 1)
2 = TCP transport on port 5000 (Serial 2)
.Set command to GC:
IR.GetDevice("Global Cache").Set("COMMAND", "")
"COMMAND" have to be created on the GC output
Example 1 (.Send and .Set command to Serial output):
var device = IR.GetDevice("Global Cache"); IR.AddListener(IR.EVENT_ONLINE , device, function() { device.Send(['POWER_ON', '\r\n'], 1); // to Serial 1 device.Send([0x00, 0x00, 0x00, 0x0D], 2); // to Serial 2 device.Set("POWER_ON", ""); // Command from the tree });
Example 2 (.Send IR command to IR Output):
var device = IR.GetDevice("Global Cache"); IR.AddListener(IR.EVENT_ONLINE , device, function() { var command1 = 'sendir,1:1,1,36127,1,1,96,31,17,16,16,16,16,32,16,32,49,32,16,16,16,16,16,16,16,16,16,16,16,16,16,16,16,16,16,16,16,16,32,17,16,16,16,16,16,32,16,16,16,16,16,16,16,16,32,32,16,16,16,16,16,16,16,16,32,17,16,16,16,16,16,32,16,4624'; device.Send([command1,0x0D]); }IR command has a following syntax:
'sendir,<connectoraddress>,<ID>,<frequency>,<repeat>,<offset>,<command sequence>'see the iTach API for details
RECEIVE FEEDBACK
To receive the feedback from Global Cache with COM port and process it with JS, please use the construction:
IR.AddListener(IR.EVENT_RECEIVE_TEXT, IR.GetDevice("Global Cache"), function(text, id) { if (id == 0) IR.Log("system data: "+ text); else if (id == 1) IR.Log("COM1 data: "+ text); else if (id == 2) IR.Log("COM2 data: "+ text); });
function (text, id)
text - the data received from equipment
id - is 0, 1 or 2, it is the number of TCP transport created by GC driver
0 = TCP transport on port 4998 (IR commands, system data, relays)
1 = TCP transport on port 4999 (Serial 1)
2 = TCP transport on port 5000 (Serial 2)
Send command to AV driver from Level or Edit-Box item
For example: $25,$50,$30,$31,xx where хх is a required volume level from 0 to 100 (in HEX format).
Another example is the ASCII string 'VALUE хх' where хх is also a required volume level from 0 to 100 (in DEC).
These values should be sent from Levels or Editbox (not from separate Buttons). It can be implemented with the help of iRidium script
Example:
function Level_ASCII() { var Packet = "VOLUME " + IR.GetItem("Page 1").GetItem("Item 6").Value + '\r\n'; IR.GetDevice("AV & Custom Systems (TCP)").Send([Packet]); };
- this function is called Level_ASCII. It can be activated with the help of the command Script Call (Level_ASCII) in the Release event of the Level item.
If your command includes a ASCII string and you should send a Value in ASCII format (for example: 'SET LEVEL XX',$0A), use this script:
// -------- to send a Volume Value from the Level var Msg = "SET LEVEL "; //This is the string with our command without volume value var Packet; IR.AddListener(IR.EVENT_ITEM_RELEASE,IR.GetItem("Page 1").GetItem("Item 1"),function() //This event will be active when you release the level-button { Packet = Msg+IR.GetVariable("Drivers.GC-100-12:Serial 1.Volume").toString()+'\n'; //Here we add the volume value to our command //IR.Log("Sended message = "+Packet) IR.GetDevice("GC-100-12:Serial 1").Send([Packet]); //here we send the result string to device });
This commands takes the slider position at the moment of its release and writes VALUE xx \r\n inside the command and then send the complete command to the AV & Custom Systems (TCP) driver.
Forming commands in НЕХ works the same way but with the data converting from DEC to HEX. Please see the example.
ASCII-HEX.irpz
Alternative sending of AV commands from one button
Selection of the Trigger button item and forming of the outgoing commands are performed in the script.
AlternateCommandSendingGC.irpz
Send a command to AV equipment automatically on project start
if this command is in the list of GC-100 Commands:
IR.AddListener(IR.EVENT_ONLINE , IR.GetDevice("GC-100-12:Serial 1"), function() { IR.GetDevice("GC-100-12:Serial 1").Set("CONNECT", ""); //IR.Log('online and sent'); });if this command is NOT in the list of GC-100 Commands:
IR.AddListener(IR.EVENT_ONLINE , IR.GetDevice("GC-100-12:Serial 1"), function() { IR.GetDevice("DEVICE").Send(['SYSTEM CONNECT', '\r\n']); //IR.Log('online and sent'); });SendCommandIfDriverOnline.irpz
AV & Custom Systems (UDP) - connection of 2 drivers
[00:00:02.418] CCustomDevice(2): StartConnect() [00:00:07.426] CCustomDevice(2): Time of waiting for connection is over! [00:00:07.441] CCustomDevice(2): StartConnect() [00:00:12.464] CCustomDevice(2): Time of waiting for connection is over!It occurs only if 2 devices communicate with the same local port.
PUT command via HTTP Driver
"GET,"
"PUT,"
"POST,"
in GUI Editor available:
"GET,"
"PUT,"
"POST,"
read example:
http://wiki2.iridiummobile.net/Drivers_API#Send
Listen to UDP packets
You have to set the IP address of broadcasting device and listen to data from it, not from all the network.
Global Cache IP2IR: problem with short commands sending
for the same one - also working with AMX devices right way. When I export codes from AMX IRL file using IRFile or Vert app, on following import do IRL file I can see, that HEX codes are OK - AMX IREdit app shows me warning, that I'm trying to import duplicate code - and it means, that are the same=OK. Discrete codes from remotecentral.com I alredy have in HEX format.
But, when I import these working HEX codes using iConvert to iRidium app, my codes are NOT WORKING with IP2IR unit... Of course, I'm using
the right method, described in manuals - one time with headers (replacing missing data - mod-addr,connaddr,repeatcount), one time
without headers (with setting: disable header = false). Only codes from GC IR database included with iRidium GUI app for SONY TV are working - but they are totally different from my, learned or downloaded from remotecentral.com and working codes!"
As I found out, problem is with Sony codes - they are too short. In GC I must repeat my code at least 2x and then it starts work. Problem is especially with Sony codes, I have confirmed this info also from Global Cache support. AMX devices send these codes by default for 0.5 second (if code is shorter,
AMX automatically repeats it), so there is difference in comparison with GC, which takes by default only one repetition and send it.
GC-100 & iTach Relay switching, triggering
You can use an item type "Trigger Button" with the value 0/1. Drag the relay command to the trigger buton with this options:
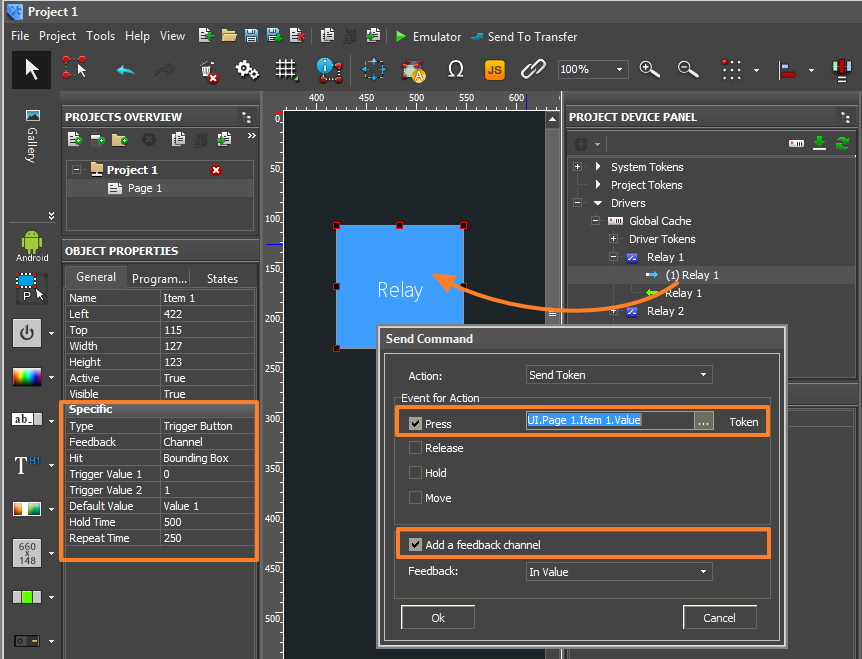
For old version of GC driver (V2.1 and older)
You can use the script module in the attachment to implement switching of GC-100 or iTach relay from the closed condition to the open one and back with the help of one button.
It monitors the current relay state and depending on it activates the command of relay closing or opening. In order to activate trigger switching you need to activate the option of monitoring the relay state and switching with the help of the ScriptCall function in macros editor. The function is assigned to the Button type graphic item.
RelayTriggerGC.irpz
RelayTriggeriTach.irpz
If the Apple TV IR codes from GC database doesn't work
If the physical remote pinned to Apple TV, you cannot control it from any other remote, the control from Global Cache doesn't work.
Customer support service by UserEcho